/***************************************\
* Financial Calculator(s) *
* March, 2005 by Harley H. Puthuff *
* Copyright 2005, Your Showcase *
\***************************************/
/*
* Global object
*/
var Our = new Object(); // global Class/Object
Our.sponsor = (typeof sponsor=='undefined') ? 'Your Showcase' : sponsor;
Our.calculator = 0; // object with calculations
Our.months = new Array('Jan','Feb','Mar','Apr','May','Jun','Jul','Aug','Sep','Oct','Nov','Dec');
Our.date = new Date(); // current date
Our.month = Our.date.getMonth()+1; // current month
Our.year = Our.date.getFullYear(); // current year
Our.round = function(v) { // round to nearest cent
return (Math.round(v*100.0)/100.0);
};
Our.roundup = function(v) { // round up to nearest cent
return (Math.ceil(v*100.0)/100.0);
};
Our.rounddown = function(v) { // round down to nearest cent
return (Math.floor(v*100.0)/100.0);
};
Our.number = function(v) { // convert to number
var p=new String(v),r=new String(''),i,d;
for (i=0;i
='0')&&(d<='9'))) r+=d;
}
if (r=='') r='0';
return Number(r);
};
Our.currency = function(v) { // convert to currency ($)
var t='',c,p;
c=(this.round(this.number(v))).toString();
p=c.split('.');
if (p.length<2) p[1]='00';
if (p[1].length==1) p[1]=p[1]+'0';
if (p[1].length>2) p[1]=p[1].substring(0,2);
if (p[0].length==0) p[0]='0';
for (c=p[0]; c.length>3;) {
t=','+c.substr(c.length-3)+t;
c=c.substr(0,(c.length-3));
}
return(c+t+'.'+p[1]);
};
Our.term = function(months,years) { // convert to "nn month(s) and nn year(s)"
var m=this.number(months),y=this.number(years),s='';
if (m>11) {y+=Math.floor(m/12); m%=12;}
if (y>0) {s+=y.toString()+' year'; if (y>1) s+='s';}
if (m>0) {if (s.length>0) s+=' and '; s+=m.toString()+' month'; if (m>1) s+='s';}
return s;
};
Our.selectDate = function() { // returns text for date selector
var m,y,t,i,j,e,yr;
m=this.month; y=this.year;
if (++m>12) {m=1; y++;}
t=' ' +
'';
return t;
};
Our.selectYears = function() { // returns text for #years selector
var i,yr,t;
t='';
return t;
};
/*
* Constructor for a Calculator object:
* P1 = loan amount or 0
* P2 = loan term (years) or 0
* P3 = loan rate (apr) or 0
* P4 = loan monthly payment or 0
* P5 = (optional) first payment month (1-12)
* P6 = (optional) first payment year (2000-????)
*/
function Calculator(name,amount,term,rate,payment,month,year,years) {
var x,y;
this.name = new String(name);
this.amount = Our.number(amount);
this.term = Our.number(term);
this.rate = Our.number(rate);
this.payment = Our.number(payment);
this.firstMonth = Our.month;
this.firstYear = Our.year;
if (++this.firstMonth > 12) {this.firstMonth = 1; ++this.firstYear;}
x = Our.number(month); if (x > 0) this.firstMonth = x;
y = Our.number(year); if (y > 0) this.firstYear = y;
this.limit = Our.number(years) * 12;
if (this.limit == 0) this.limit = this.term * 12;
if (this.term == 0) {
var mpr = this.rate / 1200;
var months = (Math.log(this.payment) - Math.log(this.payment - (this.amount * mpr))) / Math.log(1 + mpr);
this.term = Math.ceil(months / 12);
}
if (this.rate == 0) {
var months = this.term * 12.0;
var lastrate = 0.0;
var thisrate = 80.0;
var adjustment,mpr,principal;
while (1) {
adjustment = Math.abs(thisrate - lastrate) / 2.0;
mpr = thisrate / 1200.0;
principal = this.payment *
((Math.pow((1.0 + mpr), months) - 1.0) / (mpr * Math.pow((1.0 + mpr), months)));
if (Math.round(principal) == this.amount) {
this.rate = Math.round(thisrate * 100.0) / 100.0;
break;
}
lastrate = thisrate;
if (principal > this.amount)
thisrate += adjustment;
else
thisrate -= adjustment;
}
}
this.months = this.term * 12.0;
this.apr = this.rate / 100.0;
this.mpr = this.apr / 12.0;
if (this.amount == 0) {
this.amount = this.payment *
((Math.pow((1.0 + this.mpr), this.months) - 1.0) /
(this.mpr * Math.pow((1.0 + this.mpr), this.months)));
}
if (this.payment == 0) {
this.payment =
(this.amount * Math.pow((1.0 + this.mpr), this.months) * this.mpr) /
(Math.pow((1.0 + this.mpr), this.months) - 1.0);
this.payment = Our.roundup(this.payment);
}
x = (this.firstYear*12) + (this.firstMonth-1) + (this.months-1);
this.lastMonth = (x%12)+1;
this.lastYear = Math.floor(x/12);
this.numberOfPayments = this.months;
this.totalOfPayments = this.payment * this.numberOfPayments;
this.financeCharge = this.totalOfPayments - this.amount;
}
/*
* Calculator method: show mortgage/loan details
* returns text for a page with mortgage details
*/
Calculator.prototype.details = function () {
return(
'\n' +
'\n' +
'' + this.name + '\n' +
'\n' +
'\n' +
'\n' +
''
);
};
/*
* Calculator method: show amortization
* returns text for a page with amortization
*/
Calculator.prototype.amortization = function() {
var title = this.name + ' Amortization';
var row = 'even';
var text =
'\n' +
'\n' +
'' + title + '\n' +
'\n' +
'\n' +
'\n' +
'\n';
return text;
};
/*
* Evaluate the input fields and perform a calculation (if necessary)
*/
function calculate() {
var f = document.parameters,values=0;
var Amount = Our.number(f.Amount.value); if (Amount>0) ++values;
var Rate = Our.number(f.Rate.value); if (Rate>0) ++values;
var Term = Our.number(f.Term.value); if (Term>0) ++values;
var Payment = Our.number(f.Payment.value); if (Payment>0) ++values;
var Month = f.Month.selectedIndex+1;
var Year = f.Year.options[f.Year.selectedIndex].value;
var Years = f.Years.options[f.Years.selectedIndex].value;
if (Years == 0) Years = Term;
if (values < 3) {
alert('I need at least three of the four values!');
f.Amount.focus();
return;
}
if ((Amount>0) && (Rate>0) && (Term>0)) Payment=0;
Our.calculator = new Calculator('Your Mortgage',Amount,Term,Rate,Payment,Month,Year,Years);
f.Amount.value = Our.currency(Our.calculator.amount);
f.Rate.value = Our.currency(Our.calculator.rate) + '%';
f.Term.value = Math.floor(Our.calculator.term).toString() + ' years';
f.Payment.value = Our.currency(Our.calculator.payment);
results.location = "javascript:parent.Our.calculator.details()";
}
/*
* Show splash page in the results frame
*/
function splash() {
return(
'\n' +
'\n' +
'\n' +
'\n' +
'\n' + Our.sponsor + '
presents\n' +
'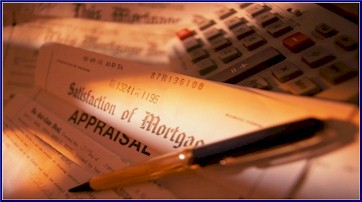
\n' +
'Your
Mortgage
Calculator
\n' +
'\n' +
''
);
}
/*
* Clear all values and restart
*/
function restart() {
var f = document.parameters,values=0;
f.Amount.value = f.Rate.value = f.Term.value = f.Payment.value = '';
Our.calculator = 0;
results.location = "javascript:parent.splash()";
f.Amount.focus();
}
/*
* insert the calculator html
*/
var d=window.document;
d.writeln('');
results.location='javascript:parent.splash()';